Enhancing Vue Applications with Internationalization (i18n)
Written on
Introduction to Internationalization in Vue
Internationalization (i18n) plays a vital role in the development of web applications aimed at a worldwide user base. Within the Vue ecosystem, the library vue-i18n simplifies the process of adding i18n capabilities to your project. This guide will walk you through the steps to integrate i18n into your Vue application, allowing you to offer a multilingual experience to your users.
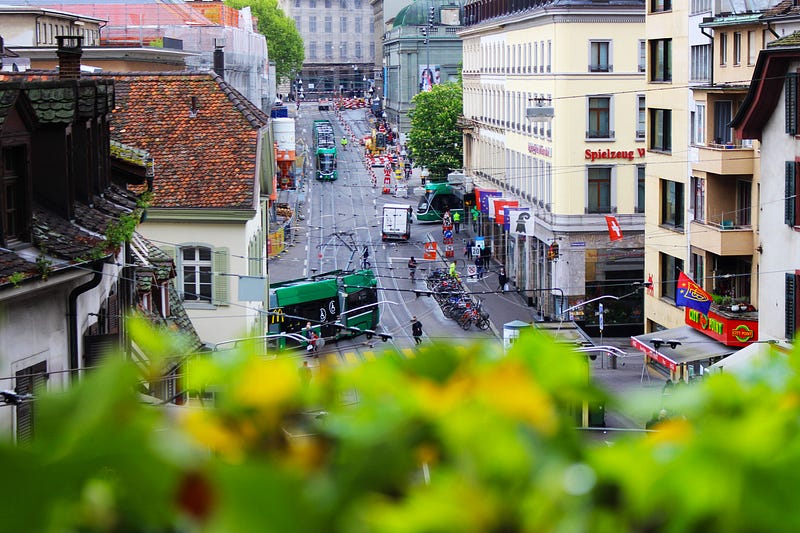
Step 1: Installing vue-i18n
First, ensure that you have a Vue project ready. If you haven't created one yet, you can easily set it up using Vue CLI. Open your terminal and navigate to your project folder, then execute the following command to install vue-i18n:
npm install vue-i18n
Step 2: Configuring vue-i18n
Create a new file called i18n.js within the src directory of your Vue project. This file will handle the configuration for vue-i18n. Begin by importing Vue and vue-i18n:
import Vue from 'vue';
import VueI18n from 'vue-i18n';
Vue.use(VueI18n);
Next, instantiate VueI18n and export it for use throughout your application:
export default new VueI18n({
locale: 'en', // default language
fallbackLocale: 'es', // fallback language
messages: {
en: {
// English translations},
es: {
// Spanish translations},
},
});
Step 3: Adding Translations
Within the messages object, input translations for each supported language. For instance, you might add the following for English and Spanish:
messages: {
en: {
greeting: 'Hello!',
welcome: 'Welcome to my application.',
},
es: {
greeting: '¡Hola!',
welcome: 'Bienvenido a mi aplicación.',
},
},
Feel free to add as many translation keys as necessary for each language.
Step 4: Organizing Translation Files
To keep your messages organized, consider creating a ‘locales’ folder for your translations.
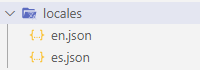
Each translation can then be stored in separate files, which you can import:
import en from './locales/en.json';
import es from './locales/es.json';
messages: {
es,
en
}
Step 5: Implementing Translations in Components
In your Vue components, import the vue-i18n instance and utilize the $t object to access translations, as shown below:
<template>
<div>
<h1>{{ $t('greeting') }}</h1>
<p>{{ $t('welcome') }}</p>
</div>
</template>
Make sure your components are set up to utilize vue-i18n by importing and assigning the vue-i18n instance in your main component:
import Vue from 'vue';
import i18n from './i18n';
new Vue({
i18n,
render: (h) => h(App),
}).$mount('#app');
Step 6: Enabling Language Switching
To provide users the option to change the language in your application, you can create a language selector or a button within your components. In the method managing the language change, employ the $i18n.locale method to dynamically switch languages:
methods: {
changeLanguage(locale) {
this.$i18n.locale = locale;},
},
Summary and Best Practices
This guide has shown you how to incorporate i18n into your Vue project using the vue-i18n library. You have configured vue-i18n, added translations, and implemented those translations in your components. Your Vue application is now equipped to handle multiple languages, providing users with a tailored experience.
Now you are prepared to develop multilingual Vue applications! Don’t forget to test and refine your translations, and feel free to explore the various features that vue-i18n offers.
If you found this article helpful, please consider following me, giving this story a clap, or subscribing to my upcoming posts. Your support is greatly appreciated!
Chapter 2: Video Tutorials on Vue i18n
Explore how to implement language translations in your Vue app with vue-i18n in this comprehensive tutorial.
Watch this video to learn how to create a language switcher in your Vue.js application, enhancing user experience with multilingual support.