Unlocking Modern JavaScript: Mastering Advanced Concepts
Written on
Chapter 1: Introduction to Advanced JavaScript
JavaScript has emerged as one of the leading programming languages, and it’s a personal favorite of mine. Its versatility in web development is remarkable, enabling the creation of interactive and responsive websites and applications. As a high-level, dynamic, and interpreted language, JavaScript possesses a variety of advanced features that are vital for developing scalable and high-performance applications. In this article, we will delve into essential advanced JavaScript concepts that every web developer should be familiar with.
Table of Contents:
- Promises
- Async/Await
- Generators
- Proxies
- Decorators
- Destructuring
- Rest and Spread Operators
- Modules
- Closures
- Memoization
- Event Loop
- Hoisting
- Arrow Functions
- Template Literals
Section 1.1: Promises
Promises are a foundational concept in JavaScript that allow developers to write cleaner, more efficient asynchronous code. They represent values that may not be immediately available but will resolve in the future, akin to real-life promises. Promises facilitate a structured approach to handle asynchronous operations.
Here’s a practical example of how to use a Promise in JavaScript:
const fetchData = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
const data = 'Hello, world!';
if (data) {
resolve(data);} else {
reject('Error: Data not found');}
}, 2000);
});
};
fetchData()
.then((data) => {
console.log(data);})
.catch((error) => {
console.error(error);});
In this code snippet, the fetchData() function returns a Promise that resolves after two seconds with the message 'Hello, world!'. The .then() method processes the resolved value, while the .catch() method handles any potential errors.
Section 1.2: Async/Await
Async/Await is a modern approach for managing asynchronous operations in JavaScript, built on top of Promises. It offers a more streamlined and readable way to write asynchronous code, making it resemble synchronous code for improved clarity.
Here’s how you can utilize Async/Await:
const getData = async () => {
try {
const data = await fetchData();
console.log(data);
} catch (error) {
console.error(error);}
};
getData();
In this example, the getData() function uses the await keyword to pause execution until the fetchData() Promise resolves. The try block captures the resolved value, while the catch block manages any errors.
Section 1.3: Generators
Generators are unique JavaScript functions that can pause and resume their execution. They enhance code efficiency and flexibility, particularly for handling complex logic and asynchronous tasks. Generators are declared using the function* syntax and utilize the yield keyword to control execution flow.
Here’s a simple example of a generator function:
function* countUpTo(max) {
let count = 1;
while (count <= max) {
yield count;
count++;
}
}
const counter = countUpTo(5);
console.log(counter.next().value); // 1
console.log(counter.next().value); // 2
In this code, the countUpTo generator yields values from 1 to the specified maximum.
Section 1.4: Proxies
Proxies in JavaScript provide an ability to define custom behaviors for object interactions. They allow developers to intercept and customize operations such as property reading, writing, and deleting, making them useful for implementing features like data validation and access control.
Here's an example of using Proxies:
const person = {
name: 'John',
age: 30,
};
const personProxy = new Proxy(person, {
get(target, property) {
console.log(Getting ${property}: ${target[property]});
return target[property];
},
set(target, property, value) {
console.log(Setting ${property} to ${value});
target[property] = value;
},
deleteProperty(target, property) {
console.log(Deleting ${property});
delete target[property];
},
});
console.log(personProxy.name); // Getting name: John
In this snippet, a Proxy object wraps the person object, enabling customized logging during property access and modification.
Section 1.5: Decorators
Decorators are a design pattern that allows the dynamic addition of behavior to objects or functions. They enable modifications without altering the original implementation, making them ideal for cross-cutting concerns like logging and validation.
Here’s a basic example of a decorator:
class Component {
render() {
return 'Component';}
}
class Decorator {
constructor(component) {
this.component = component;}
render() {
return Decorated(${this.component.render()});}
}
const component = new Component();
const decoratedComponent = new Decorator(component);
console.log(decoratedComponent.render()); // Decorated(Component)
This code demonstrates how the Decorator class adds additional behavior to the Component class's render() method.
Chapter 2: Advanced Features
Section 2.1: Destructuring
Destructuring is a powerful ES6 feature that simplifies the extraction of values from arrays and objects. It enhances code readability and conciseness.
const numbers = [1, 2, 3, 4, 5];
const [first, second, , fourth] = numbers;
console.log(first); // Output: 1
console.log(second); // Output: 2
console.log(fourth); // Output: 4
In this example, destructuring allows for the straightforward assignment of array values to variables.
Section 2.2: Rest and Spread Operators
The rest (...) and spread (...) operators are related features that provide powerful ways to manage arrays and objects.
Rest Operator Example:
function sum(...numbers) {
return numbers.reduce((acc, num) => acc + num, 0);
}
console.log(sum(1, 2, 3, 4, 5)); // Output: 15
Spread Operator Example:
const originalArray = [1, 2, 3, 4, 5];
const clonedArray = [...originalArray];
console.log(clonedArray); // Output: [1, 2, 3, 4, 5]
These operators enhance the ability to work with functions and data structures flexibly.
Section 2.3: Modules
Modules are a method for structuring and encapsulating code in separate files, promoting better organization and reusability. They use import and export statements to define and use modules.
Module Example:
math.js:
export const add = (a, b) => a + b;
export const subtract = (a, b) => a - b;
app.js:
import { add, subtract } from './math.js';
console.log(add(2, 3)); // Output: 5
Using modules fosters cleaner code organization in JavaScript applications.
Section 2.4: Closures
Closures are a robust feature in JavaScript that allows functions to retain access to their lexical scope, even after the outer function has executed. This facilitates encapsulation and private data management.
Here’s an illustrative example:
function makeAdder(x) {
return function(y) {
return x + y;}
}
const add5 = makeAdder(5);
console.log(add5(3)); // Output: 8
In this case, the add5 function retains access to the variable x from its closure.
Section 2.5: Memoization
Memoization is a technique to optimize function performance by caching results for specific inputs. This avoids redundant computations, enhancing execution speed.
Here’s how memoization works:
const fibonacci = (n) => {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
};
const memoize = (fn) => {
const cache = new Map();
return function(n) {
if (cache.has(n)) return cache.get(n);
const result = fn(n);
cache.set(n, result);
return result;
}
}
const memoizedFibonacci = memoize(fibonacci);
console.log(memoizedFibonacci(50)); // Output: 12586269025
This example illustrates how memoization can significantly improve performance for computationally heavy functions.
Section 2.6: Event Loop
The Event Loop is a fundamental component of JavaScript that manages asynchronous behavior, ensuring non-blocking execution of tasks.
Understanding the Event Loop is crucial, especially when working with asynchronous operations like timers, promises, and callbacks. Here’s a quick demonstration of how it operates:
console.log('Start');
setTimeout(() => {
console.log('Timeout 1');
}, 0);
setTimeout(() => {
console.log('Timeout 2');
}, 1000);
console.log('End');
In this example, the synchronous console.log statements execute first, followed by the asynchronous setTimeout callbacks.
Section 2.7: Hoisting
Hoisting refers to JavaScript's behavior of moving variable and function declarations to the top of their containing scope during compilation. This can lead to unexpected outcomes if not understood properly.
Variable Hoisting Example:
console.log(x); // Outputs: undefined
var x = 10;
Function Hoisting Example:
foo(); // Outputs: "Hello, world!"
function foo() {
console.log("Hello, world!");
}
To avoid confusion, it’s advisable to declare variables at the top of their scope and utilize function declarations where necessary.
Section 2.8: Arrow Functions
Arrow functions, introduced in ES6, provide a more concise syntax for writing functions in JavaScript. They allow for cleaner code and have unique behavior, especially regarding the this keyword.
Arrow Function Example:
const add = (a, b) => a + b;
console.log(add(2, 3)); // Output: 5
Arrow functions can simplify coding and enhance readability.
Section 2.9: Template Literals
Template Literals offer a more readable way to create strings in JavaScript, allowing for easy interpolation of variables and expressions.
Template Literal Example:
const name = "John";
const age = 30;
const message = My name is ${name} and I am ${age} years old.;
console.log(message); // Output: My name is John and I am 30 years old.
They enhance code clarity and facilitate multi-line strings.
Conclusion
This guide on mastering advanced JavaScript concepts aims to equip you with the knowledge needed to leverage these features in your projects. Understanding these advanced topics will undoubtedly enhance your coding skills and improve your web development capabilities.
Follow me on Twitter for more insights.
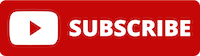