Vue 3 Development: Integrating PrimeVue Dropdown and Editor Components
Written on
Chapter 1: Introduction to PrimeVue
PrimeVue is a user interface framework designed to work seamlessly with Vue 3. In this guide, we'll explore how to effectively develop Vue 3 applications using the PrimeVue library.
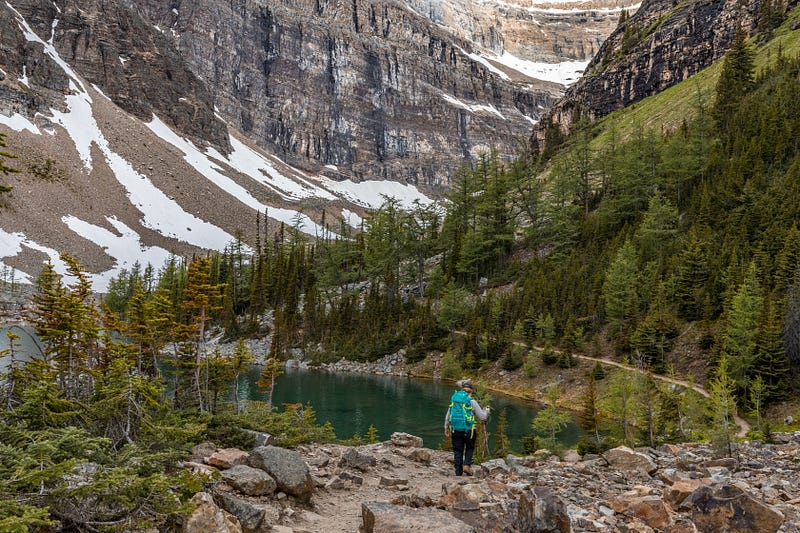
Chapter 2: Implementing Dropdown in Vue 3
To incorporate a dropdown menu into our Vue 3 application, we can utilize the Dropdown component provided by PrimeVue. Below is an example of how to set this up:
// main.js
import { createApp } from "vue";
import App from "./App.vue";
import PrimeVue from "primevue/config";
import Dropdown from 'primevue/dropdown';
import 'primevue/resources/primevue.min.css';
import 'primevue/resources/themes/saga-blue/theme.css';
import 'primeicons/primeicons.css';
import 'primeflex/primeflex.css';
const app = createApp(App);
app.use(PrimeVue);
app.component("Dropdown", Dropdown);
app.mount("#app");
In your App.vue, you can create a dropdown like this:
<template>
<div>
<Dropdown
v-model="selectedCity" :options="cities"
optionLabel="name"
placeholder="Select a City"
/>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
selectedCity: "",
cities: [
{ name: "New York", code: "NY" },
{ name: "Miami", code: "MI" },
{ name: "London", code: "LDN" },
],
};
},
};
</script>
Here, the name property is shown to the user as indicated by the optionLabel prop, while the code serves as the value. The placeholder appears as a prompt in the dropdown.
To enhance user experience, the filter property allows users to search through the options:
<template>
<div>
<Dropdown
v-model="selectedCity" :options="cities"
optionLabel="name"
placeholder="Select a City"
filter
/>
</div>
</template>
Additionally, you can customize how each choice and the selected value are displayed using slots:
<template>
<div>
<Dropdown
v-model="selectedCity" :options="cities"
optionLabel="name"
placeholder="Select a City"
showClear
>
<template #value="slotProps">
<div class="p-dropdown-code">
<span v-if="slotProps.value">{{ slotProps.value.name }}</span>
<span v-else>{{ slotProps.placeholder }}</span>
</div>
</template>
<template #option="slotProps">
<div class="p-dropdown-name">
<span v-if="slotProps.option">{{ slotProps.option.name }}</span>
<span v-else>{{ slotProps.placeholder }}</span>
</div>
</template>
</Dropdown>
</div>
</template>
Chapter 3: Adding a Rich Text Editor
To introduce a rich text editor in our Vue 3 application, we can leverage PrimeVue's Editor component, which is built on the Quill rich text editor framework. Start by installing the Quill package:
npm i quill
Then, set it up in your application:
// main.js
import { createApp } from "vue";
import App from "./App.vue";
import PrimeVue from "primevue/config";
import Editor from 'primevue/editor';
import 'primevue/resources/primevue.min.css';
import 'primevue/resources/themes/saga-blue/theme.css';
import 'primeicons/primeicons.css';
import 'primeflex/primeflex.css';
const app = createApp(App);
app.use(PrimeVue);
app.component("Editor", Editor);
app.mount("#app");
In App.vue, the editor can be added like this:
<template>
<div>
<Editor v-model="value" editorStyle="height: 320px" /></div>
</template>
<script>
export default {
name: "App",
data() {
return {
value: "",};
},
};
</script>
The editorStyle prop allows you to define the editor's dimensions, and the content typed into the editor is linked to the value reactive property via v-model.
Conclusion
In summary, integrating both a dropdown and a rich text editor into your Vue 3 application is straightforward using the PrimeVue framework. With these components, you can significantly enhance the user interface of your web applications.
Chapter 4: Video Tutorials
Explore the process of starting with Vue 3 and PrimeVue in this introductory video.
Learn about PrimeVue as a comprehensive UI library for the Vue ecosystem in this detailed video.