Mastering Java: Top 10 Coding Practices for Better Code
Written on
Chapter 1: Introduction to Java Best Practices
Java is a popular programming language appreciated for its ease of use and adaptability. However, to ensure that your code is both efficient and maintainable, adhering to best practices is crucial. Below are ten essential practices for writing Java code effectively.
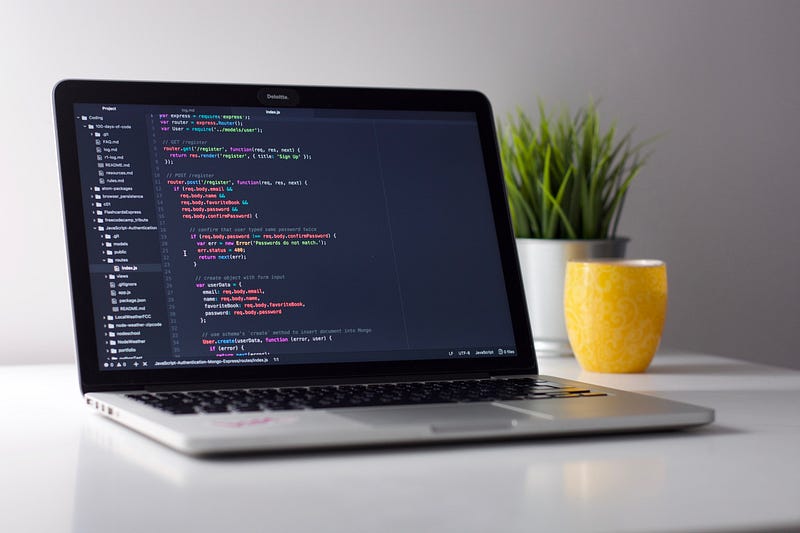
Section 1.1: Meaningful Naming Conventions
Utilize descriptive names for variables, methods, and classes. This approach enhances the readability of your code, making it easier for others (and yourself) to understand your intent.
Section 1.2: Keep Methods Concise
Long methods can be challenging to comprehend and maintain. Aim to create shorter, more focused methods that accomplish specific tasks.
Section 1.3: Implement SOLID Principles
The SOLID principles guide effective object-oriented design. They promote practices such as single responsibility, open-closed, Liskov substitution, interface segregation, and dependency inversion, all of which contribute to better software architecture.
Section 1.4: Favor Interfaces Over Concrete Classes
By using interfaces, you define contracts that various classes can implement, enhancing flexibility and simplifying future modifications.
Chapter 2: Handling Nulls and Resources
Section 2.1: Avoid Null Values
Using null values can introduce runtime errors and complicate code comprehension. Prefer the Optional class or other null-safe alternatives to manage potential nulls effectively.
Section 2.2: Utilize Try-With-Resources
The try-with-resources statement allows automatic closure of resources, eliminating the need for explicit try-finally constructs, thereby simplifying resource management.
Section 2.3: Limit Global and Static Variables
Global and static variables can create tight coupling among classes, complicating maintenance. Strive to minimize their use for cleaner code architecture.
Chapter 3: Exception Handling and Logging
Section 3.1: Appropriate Use of Exceptions
Exceptions should signal unusual conditions rather than serve as a control mechanism. Use them judiciously to maintain clear and effective error handling.
Video Description: Unlock the secrets of Java coding with this video on best practices that can elevate your programming skills to a professional level.
Section 3.2: Implement Logging Frameworks
Logging frameworks enable you to record messages at various levels, aiding in diagnosing issues within your application.
Chapter 4: Design Patterns in Java
Section 4.1: The Importance of Design Patterns
Design patterns provide tried-and-true solutions to common programming challenges. They help structure your code, making it easier to understand and maintain.
Video Description: Discover the first in a series of good coding practices with a focus on competitive programming in Java.
By adhering to these best practices, you can produce Java code that is efficient, maintainable, and comprehensible. While these guidelines are generally advisable, remember that there may be instances where you need to deviate from them to meet specific requirements or improve performance. Strive to balance readability, performance, and maintainability in your coding endeavors.
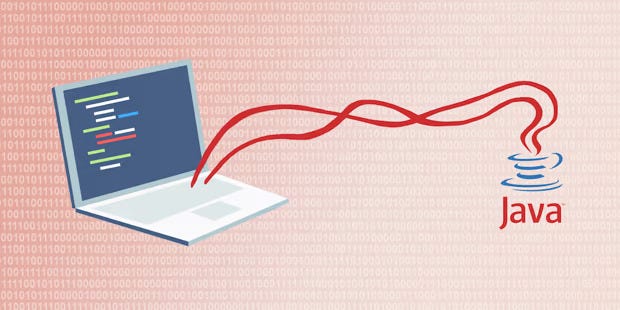