Beginner's Guide to Mockito Unit Testing with JUnit 5
Written on
Chapter 1: Understanding Mockito and Unit Testing
Unit testing is a crucial practice to ensure your code behaves as intended. Often, the classes requiring tests are reliant on other classes, making it challenging to test them directly. In such cases, the Mockito library provides a solution by allowing us to mock the dependent classes, enabling a focus on the class under test. This guide delves into the use of Mockito and its basic annotations for writing unit test cases.
Section 1.1: A Practical Example
Consider three classes: ClassA, ClassB, and ClassC, where ClassC is our focus for testing. ClassC's functionality depends on ClassA and ClassB. We will evaluate a method named combineString within ClassC.
public class ClassC {
private final ClassA classA;
private final ClassB classB;
public ClassC(ClassA classA, ClassB classB) {
this.classA = classA;
this.classB = classB;
}
protected String combineString() {
final String str1 = classA.getSomeString();
final String str2 = classB.getSomeString();
final StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append(str1);
stringBuilder.append(" and ");
stringBuilder.append(str2);
return stringBuilder.toString();
}
}
This method retrieves a string from each of the dependent classes and concatenates them using a StringBuilder. Let's proceed to the setup.
Section 1.2: Setting Up Dependencies
In addition to JUnit 5, you will need to include the following dependencies for Mockito:
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>4.6.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-junit-jupiter</artifactId>
<version>4.6.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.2</version>
<scope>test</scope>
</dependency>
Make sure to use the latest versions available.
Section 1.3: Initializing the Test Class
We begin by annotating our test class with @ExtendWith(MockitoExtension.class). This annotation enables us to utilize Mockito's capabilities within our test class. The dependencies, ClassA and ClassB, will be mocked and defined as fields, while ClassC will be instantiated normally.
@ExtendWith(MockitoExtension.class)
public class SampleClassCTest {
@Mock
private ClassA classA;
@Mock
private ClassB classB;
private ClassC classC;
@BeforeEach
void setup() {
classC = new ClassC(classA, classB);}
}
Section 1.4: Writing Test Cases
Now we can write our unit test. The following example verifies that the result from combineString begins with the string from ClassA and concludes with the string from ClassB. Since we are using mocks, the getSomeString method will not return actual values. We can define return values using the when...thenReturn... syntax in Mockito.
@Test
public void testCombineString() {
final String str1 = "TestStringA";
final String str2 = "TestStringB";
when(classA.getSomeString()).thenReturn(str1);
when(classB.getSomeString()).thenReturn(str2);
final String result = classC.combineString();
Assertions.assertEquals(true , result.startsWith(str1));
Assertions.assertEquals(true, result.endsWith(str2));
}
In this snippet, we create two test strings and configure our mock classes to return these values when their methods are invoked. When combineString is called, the method will behave according to the specified return values for getSomeString.
Section 1.5: Running the Test
The test case has successfully passed. You can now proceed to add additional test cases using similar methodologies.
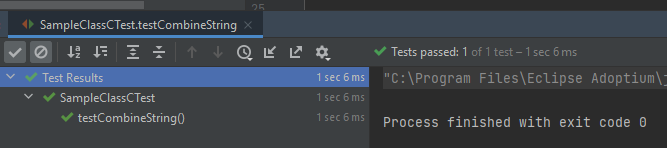
I hope you find this guide informative. If you're eager to expand your knowledge in technology or seek inspiration from daily experiences, feel free to follow my channel for updates.
Chapter 2: Video Tutorials
To enhance your understanding, here are some recommended video resources:
The first video titled "Complete JUnit & Mockito Tutorial Course: From Zero to Hero 2022" provides an in-depth look at unit testing with JUnit and Mockito. This comprehensive course is perfect for beginners and seasoned developers alike.
The second video, "Mockito Tutorial - Mocking With Junit and Maven," focuses on mocking techniques using Mockito in conjunction with JUnit and Maven. This tutorial is ideal for those looking to deepen their understanding of effective testing strategies.